Python Study Notes - File Operations
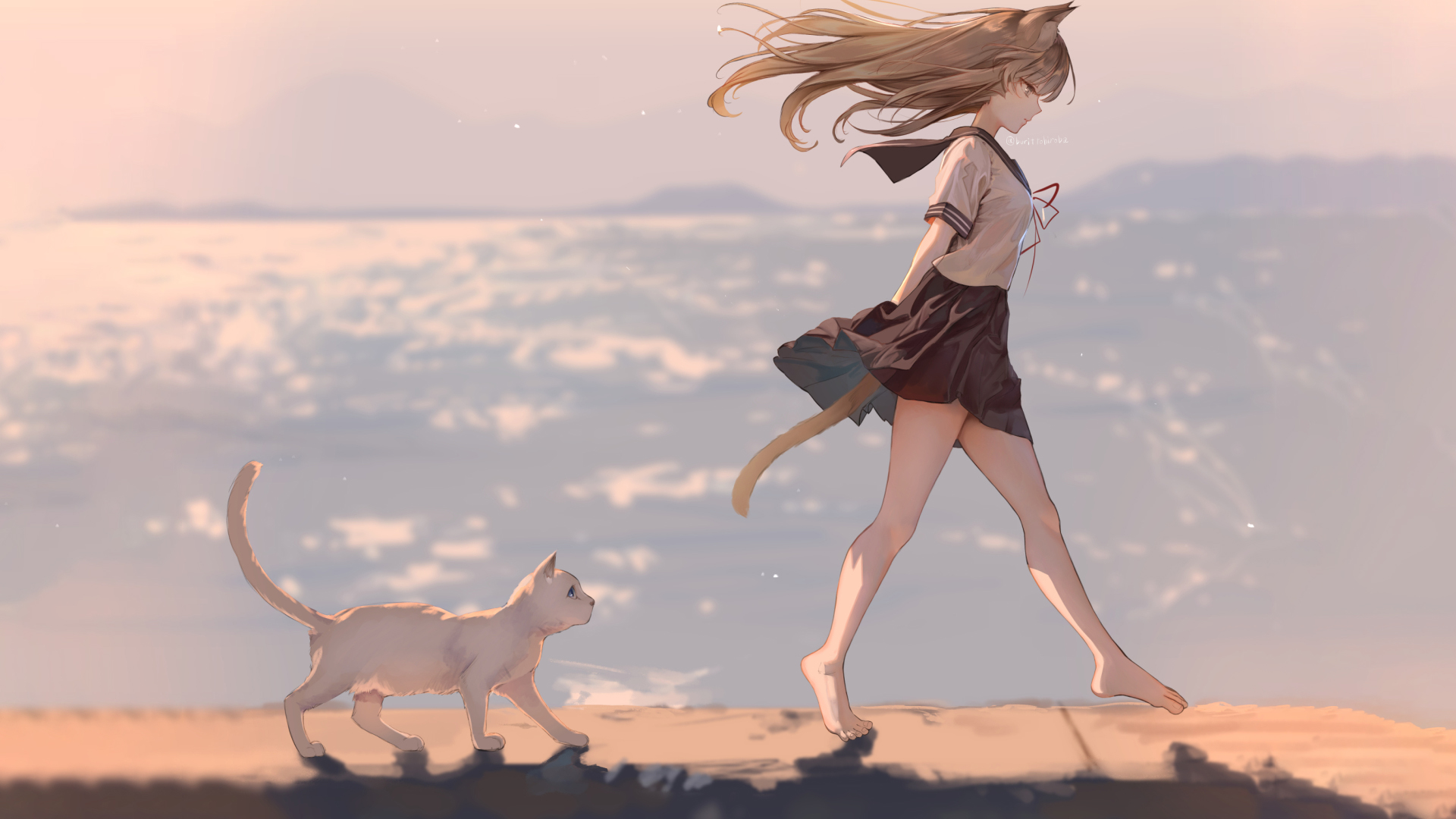
File Operations
open
open()
is the key function in Python for file operations, with two parameters that need to be set:
- Filename - the name of the file, self-explanatory
- Mode - determines if the file being opened can be read/written to or has other attributes.
|
|
Reading
Open a file in read-only mode:
|
|
This is equivalent to:
|
|
“r” indicates to read
“t” indicates that the file is text, this is the default setting for the function, so it can be omitted.
Here’s a list from w3schools:
There are four different methods (modes) for opening a file:
“r” - Read - Default value. Opens a file for reading, error if the file does not exist
“a” - Append - Opens a file for appending, creates the file if it does not exist
“w” - Write - Opens a file for writing, creates the file if it does not exist
“x” - Create - Creates the specified file, returns an error if the file exists
In addition you can specify if the file should be handled as binary or text mode
“t” - Text - Default value. Text mode
“b” - Binary - Binary mode (e.g. images)
For example, let’s say we have a file:
|
|
We can read the file without specifying the mode parameter:
|
|
Output:
|
|
Or we can specify it:
|
|
Output:
|
|
Reading lines
File:
|
|
When we encounter a multiline file, we can choose to read only one line at a time using f.readline()
For example:
|
|
Output:
|
|
If we need two lines:
|
|
Output:
|
|
If we need three lines:
|
|
Output:
|
|
This usage reads line by line and prints with line breaks.
You may need it when reading configuration files…
Of course, we can also use a for()
loop to read all lines at once:
|
|
Output:
|
|
I think using for
is more efficient…
Closing files
Nothing much to say here…
|
|
The effect is similar to the previous example.
I won’t provide debugging results below, it’s too late.
Creating
“x” indicates creating a new file. If the specified filename already exists, an error will be returned.
|
|
Try it out yourself, nothing much else.
Writing to a file
The character 'a'
represents adding content to an existing file without deleting or overwriting its original contents.
For example:
|
|
The above string will be added to the file.
The character 'w'
represents overwriting the file, which will replace any existing content.
For example:
|
|
In this case, only the string specified will exist in the file.
Deleting a file
You need to use the os
module and its os.remove()
function. Simply type import os
to import it.
|
|
Classic example
Check if a file exists, delete it if it does, or display a message if it doesn’t.
Deleting a directory
Use os.rmdir()
.
|
|
Conclusion
These are the basics of file read/write operations that you should know.
If you’re having trouble understanding, you can try running the following code with different open()
mode parameters.
Summary code:
|
|